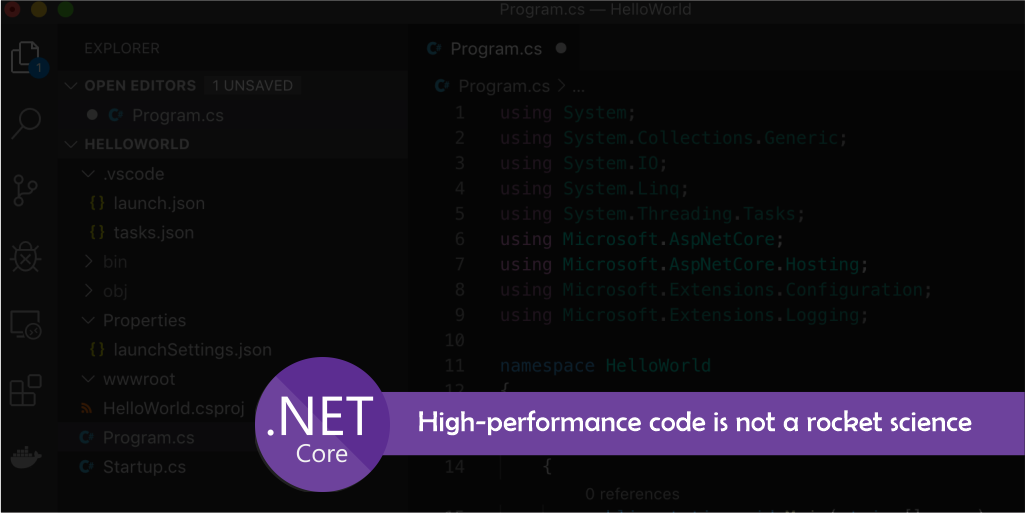
Writing a high performance code is not a rocket science
When we talk about high-performance code, we always think “it is very difficult and only person with a lot of experience can write such code”. But this is wrong perception. Here are some simple tricks and tips to write high-performance code. (Note: Here, I have taken examples of C# language and SQL Server database.)
Never perform database operation inside loop (unless and until it is unavoidable)
I have seen many code that use looping for database operation unnecessarily.
Take a look at below code:
The code shown in above Figure 1 and Figure 2 is example of bad practice of looping with database operation. This can lead to very low performance of any application because on every call of AddEmployee function, new connection to the database will be created and then new record will be inserted in database.
In contrary, let’s check below code:
Instead of saving record one-by-one in database, we can save the records in bulk in database in one shot as shown in Figure 3 and Figure 4. This can give boost in performance of application. (Note: Here, AddRange is inbuild function of LINQ.)
Database: inner query v/s join query
Now, let’s check below two examples of database query. Figure 5 is example of query with inner query (sub query) and Figure 6 is example of query with inner join.
Although the result of both the above queries will be same in terms of records, there is huge difference in performance of both the queries. Joins will execute query faster than sub query. Please check below statistics for execution of both the queries performed on table with approximately 550 records.
Figure 7 is statistics of query with inner query (sub query). Figure 8 is statistics of query with join query. We can see that join query executes in 5 milliseconds and inner query executes in 30 milliseconds. This difference can increase on tables with large amount of data
DataTable v/s Dictionary
When you need to perform filter on data based on some fix value, use Dictionary in place of DataTable. Let’s check with below example:
Data filtering with Dictionary is very fast as compared with DataTable. In Figure 9 dtEmployee is DataTable. In Figure 10 dicEmployee is Dictionary that has EmployeeCode as key. There are 500 records each in DataTable and Dictionary. In above example, Dictionary will improve performance of data filtering. We can see statistics in below image.
With approximately 500 records, data filtering with DataTable takes 14 milliseconds and data filtering with Dictionary takes 0 milliseconds.
Keep variable and object scope in mind
When we declare any variable/object, we should always keep its scope in our mind. Variables declared at wrong place can lead to memory leakage and ultimately results in low performance of application. We should follow proper guideline for variable/object declaration and use.
- Declare variable at function level, if it is going to use only in that function.
- Don’t declare any variable at global level unless and until it is going to be used at application level.
- Try to declare variables with proper data type (don’t use var if data type of variable is known).
- Use proper access modifiers (public, private, protected, etc.) to restrict access of variable/object at certain level.
- Try to declare and use objects in Using code block. With Using, the memory occupied by that object will be disposed automatically after code exits Using block.
Cache your static data
Every application has some static data that is not going to change frequently. For example, Country, State, City, Gender, Name initials, and many more. We can cache those data using any of caching method. When we need to fetch those data, we can retrieve those data from cache instead of querying in database. This will boost application performance. Whenever these is any change in those cached data, we can update those data in cache as well.
Basically, there are three types of cache: In-memory cache, Persistent in-process cache and Distributed cache. You can choose caching type as per your requirement.
You can choose from the following cache providers as per your selected type of cache:
- Memory Cache
- Redis
- OrmLiteCacheClient
- Memcached
- Aws DynamoDB
- Azure Table Storage
Conclusion
These are very few points that can be considered when writing a code for high-performance application. But one can always start by small things and gradually can increase performance of any application.